Spring-EL
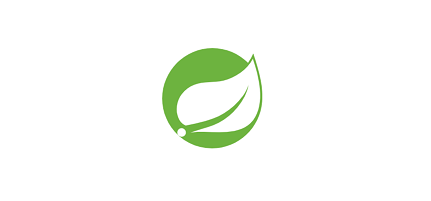
Spring-EL
cmyangSpringEL实现规则表达式判断
Spring 表达式语言(简称“SpEL”)是一种强大的表达式语言
1 | public static void main(String[] args) { |
项目中动态计算表达式值的过程
从页面上配置表单式,左值选择表单中的属性或者接口返回对象属性,中间选择比较符号,右值选择枚举或者输入字符串
使用时,生成完整表达式,并获取计算表达式需要的所有的数据
1
(#fun1([formModules][MD_OC], "[status]=='1'") && [formModules][MD_PROJECT][projectStatusId]=='13123b02-74a6-4ec4-8978-77ec446d4e29') || (#fun1([formModules][MD_OC], "[status]=='1'") && [formModules][MD_PROJECT][projectStatusId]=='3619a136-7988-440a-84d1-ccf8a7e2cb06')
通过SpringEL模板引擎计算结果
1 | /** |
引用的方法主要处理一些内置表达式无法完成的规则计算,这里是为了计算接口返回的集合中的每一条数据是否都满足条件
1 | public static boolean fun1(List<Object> arr, String exp) { |
SpEL支持的功能
从对象,集合,Map中获取数据
从对象获取数据
1
2
3
4
5
6
7
8public static void main(String[] args) {
User user = new User("张三");
EvaluationContext evaluationContext = new StandardEvaluationContext(user);
ExpressionParser parser = new SpelExpressionParser();
Expression exp = parser.parseExpression("#root.name");
String message = exp.getValue(evaluationContext, String.class);
System.out.println(message); //张三
}从集合获取数据
1
2
3
4
5
6
7
8public static void main(String[] args) {
List<User> users = Arrays.asList(new User("张三"), new User("李四"), new User("王五"));
EvaluationContext evaluationContext = new StandardEvaluationContext(users);
ExpressionParser parser = new SpelExpressionParser();
Expression exp = parser.parseExpression("#root[2].name");
String message = exp.getValue(evaluationContext, String.class);
System.out.println(message); //王五
}从Map获取对象
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15public static void main(String[] args) {
Map<String, Object> map = new HashMap<>();
map.put("name", "张三");
map.put("age", 18);
Map<String, Object> detail = new HashMap<>();
detail.put("address", "合肥");
map.put("detail", detail);
EvaluationContext evaluationContext = new StandardEvaluationContext(map);
ExpressionParser parser = new SpelExpressionParser();
String express = "'姓名:' + [name] + ',年龄:' + [age] + ',地址:' + [detail][address]";
Expression exp = parser.parseExpression(express);
String message = exp.getValue(evaluationContext, String.class);
System.out.println(message); //姓名:张三,年龄:18,地址:合肥
}
判空处理
Map的判空操作
如果在StandardEvaluationContext中设置的对象是一个map,在判空获取值时,不能使用[xxx]这种方式,需要使用get方式
例如:从approve中获取summary参数值,approve有可能为null,summary也有可能为空
1
'摘要:'+(#root.get('approve')?.get('summary')?:'')
计算符号
支持基本的运算符,括号内和符号是等价的
1
2
3
4
5
6
7
8
9
10
11
12lt( < ), gt( > ), le( <= ), ge( >= ), eq( == ), ne( != ), div( / ), mod( % ), not( ! )
and( && ), or( || ), not( ! )
+, -, *, /, %, ^
instanceof
parser.parseExpression("'xyz' instanceof T(Integer)").getValue(Boolean.class);
matches
parser.parseExpression("'5.00' matches '^-?\\d+(\\.\\d{2})?$'").getValue(Boolean.class);
函数调用
可以调用java的方法
1 | public static void main(String[] args) { |
自定义函数
当基本的运算符和方法无法满足表达式计算时,可以自定义函数,在表达式中调用自定义函数
1 | public class ELExpress { |